Program examples using the Imec sensing engine API
The Imec sensing engine is programmed in C, and can be set-up, configured and used with the sensing API. Using a simple example the use of this API is illustrated for the FFT_SWEEP mode. Both a single FFT sweep as multiple FFT sweeps are shown. This is illustrated for an Imec sensing engine equiped with a WARP front-end. A zip archive containing the source code of the examples is included below.
To conclude we provide an overview of the other modes as supported by the Imec sensing engine.
FFT_SWEEP example
The Imec sensing engine supports several modes: for this example uses FFT_SWEEP sensing scheme. This returns 128 points per selected channel. Figure 1 shows the output of one FFT sweep, for channel one to four for WARP, covering center frequencies 2412, 2432, 2452, 2472.
Figure 1 Output of one FFT sweep in the ISM band
The sensing engine is programmed in C. Items (1) .. (8) show the necessary steps to configure the sensing engine, and to produce the data shown in Figure 1. The full source code is available in the zip archive, specifically in the warp_single_FFT_SWEEP.c program file. In (9) we show how the program can be extended to do multiple sweeps.
The next paragraph discuss the required calls to the sensing API to produce the result.
Convention:
Type declarations are highlighted in green, and code snippets in blue. Calls to the sensing engine API are underlined.
1) Open the sensing engine handler
To open an Imec sensing engine board both the spider number and
front end number need to be used. The output is a software handler
that is used for the sensing engine API function calls.
se_t sensing_engine_handler;
sensing_engine_handler = se_open(spider, warp);
With:
- spider: an integer containing the spider board number. This number is bigger than 128 for spider v2 boards
- warp: a constant value 0 to select the WARP front end.
2) Initialize sensing engine
After checking the status of the sensing engine, the se_init function is
invoked to allocate memory and start adres for the my_se_config struct.
With this struct the parameters for the sensing engine will be configured.
A return value equal to 1 indicates that this step succeeded
int result = 0;
struct se_config_s my_se_config;
// configure WARP FFT sweep
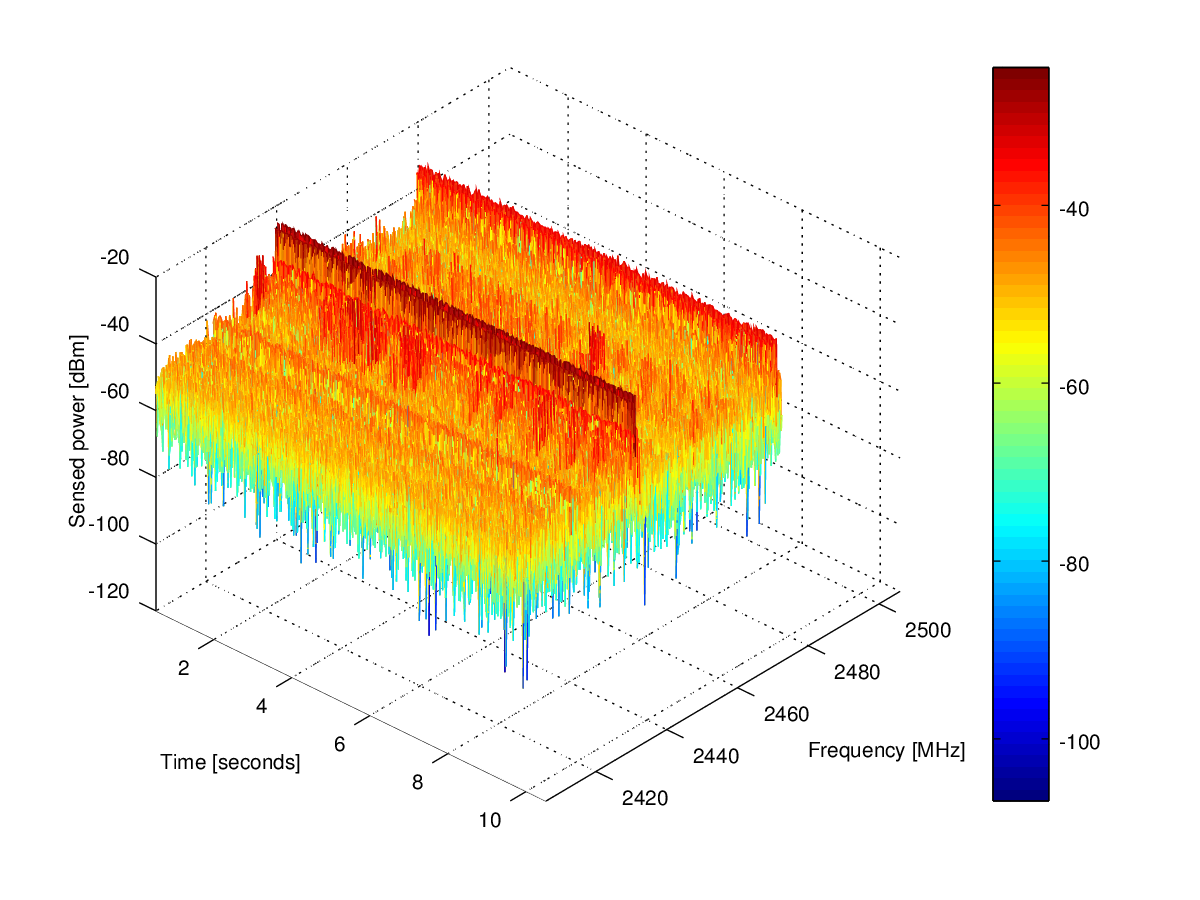
Other sensing modes
- FFT_SWEEP : shown in example above. A 128 point FFT is performed for each configured channel.
- WLAN_G: determines the instantaneous power in each selected channel for IEEE802.11g spectrum.
- WLAN_A: similar as WLAN_G, for IEEE802.11a spectrum.
- BLUETOOTH: determines instantanious power for IEEE802.15.1 spectrum.
- ZIGBEE: similar to BLUETOOTH, for IEEE802.15.4 spectrum
- LTE: not implemented.
- DVB_T: detection of DVB_T signals. Only applicable for the Scaldio2B front-end.
- ISM_POWER_DETECT: determines instantaneous power in the 2.4 GHz ISM band, with a granularity of 1 MHz.
- TRANSMIT: not implemented.
- ADC_LOG1: logging of ADC values as retrieved from the front-end. Debug mode for sensing engine equiped with a Scaldio2B front-end.
- ADC_LOG2: logging of ADC values as retrieved from the front-end. Debug mode for sensing engine equiped with a WARP front-end.
- STANDBY: not implemented.
Attachment | Size |
---|---|
WARPexample.zip | 5.62 KB |
Imec-sensing-engine-WARP-single-FFT-sweep.png | 10.84 KB |
Imec-sensing-engine-WARP-multiple-FFT-sweep.png | 324.93 KB |